Objective
The objective of Milestone 2 was to make the robot successfully avoid walls and use right-hand turn following. To do this, we needed to integrate IR sensors and have LEDs to indicate robot "thinking".
Materials
- 3 IR sensors
- 1 blue LED
- 1 red LED
- 1 white LED
- Breadboard
Hardware
IR Sensors
We mounted short-range IR sensors on the front, right, and left of the robot. These IR sensors were very easy to wire, as they only had a power, ground, and input pin each. We had to solder extra wires to the ends of the IR sensors, as the wires were very thin.
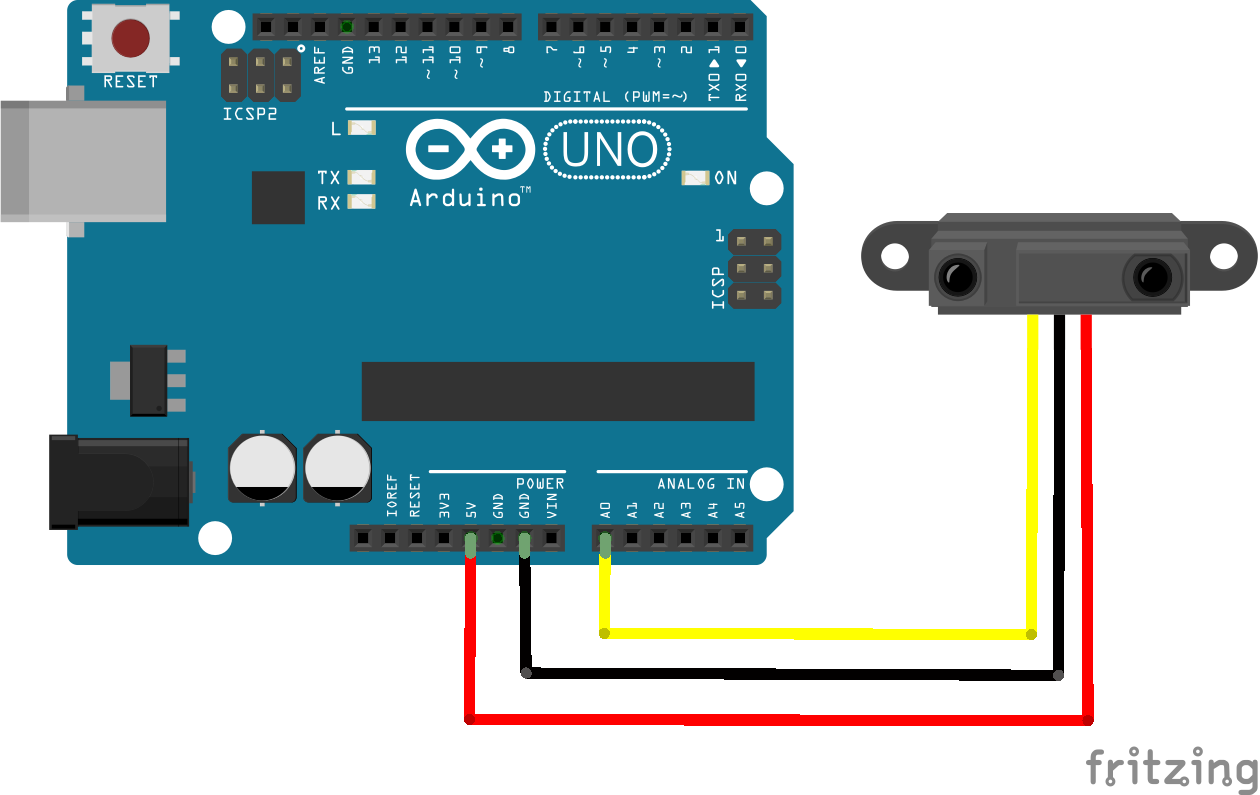
Robot Redesign
We decided to clean up the robot, as a lot of the components from earlier labs/milestones were on different breadboards and had very bad wiring. We first decided to make the wiring tighter to the board so the robot looked cleaner. This made the robot more organized and opened up more space on the breadboard. We then integrated the Schmitt Triggers onto the robot, as it was separate before. On a different breadboard, we replicated the circuit three times for each of the line sensors. Note that we disconnected the microphone input to its analog pin, but implementing the Schmitt Triggers also freed up more analog pins such that we can reconnect it later.
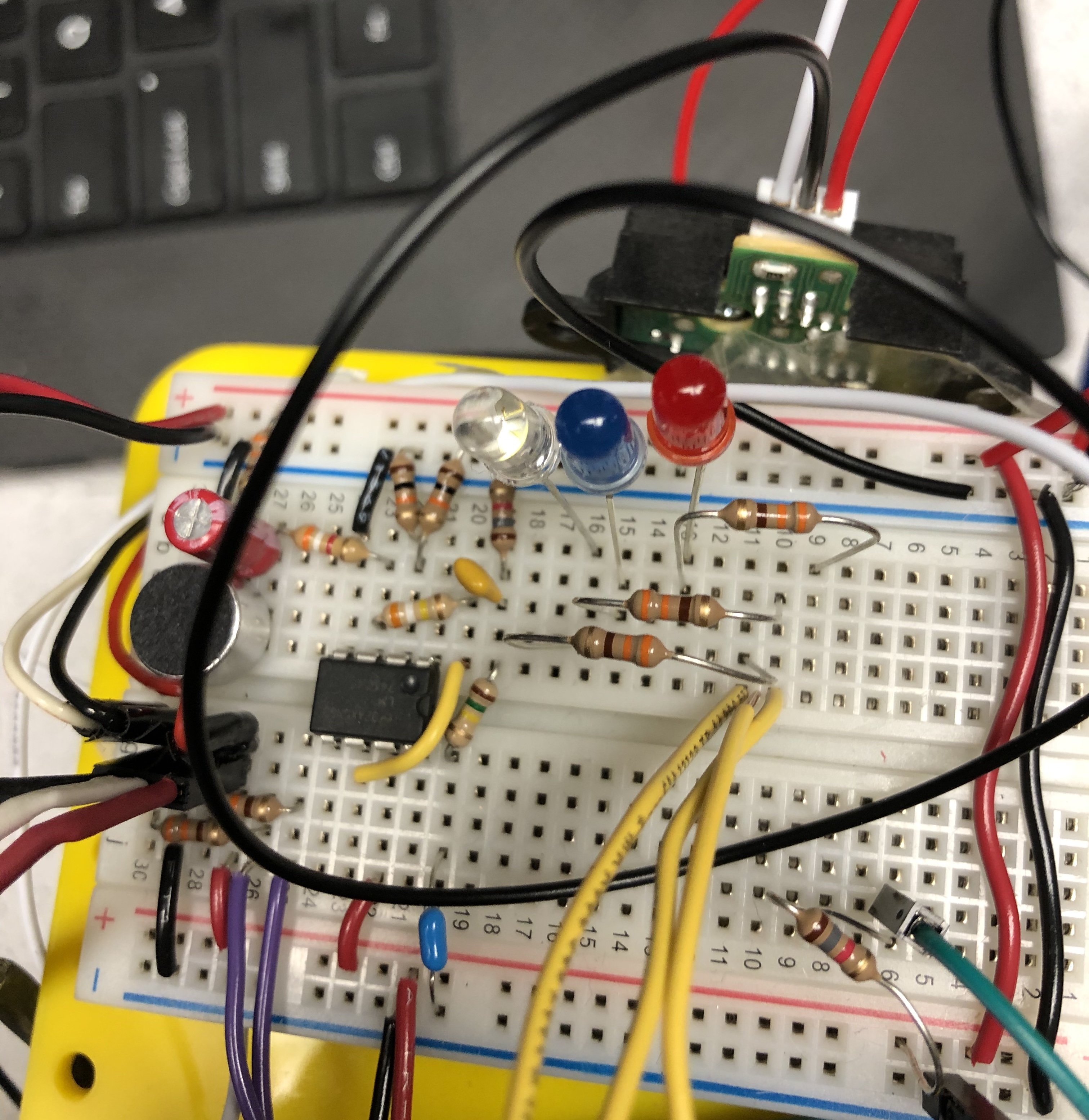
Software
Wall Sensing
The IR sensors give us a voltage, so we needed some way to convert voltage to distance. Looking online, we found that the distance conversion required the following code, assuming that "sensor" is the output from the IR sensor.
float volts = analogRead(sensor)*0.0048828125; // value from sensor * (5/1024) int distance = 13*pow(volts, -1);
After getting this distance, we needed to experimentally determine a good threshold for distance from robot to wall. We determined a distance of 20 was sufficient to successfully detect the wall. We also included 3 LEDs to the robot that would light up depending on what wall the robot sensed (red for right wall, white for front wall, blue for left wall). These LEDs were independent of the wall sensing logic and instead were tied directly to the IR sensor readings. These LEDs were put in series with 330Ω resistors and can be seen in the above picture.
Right-hand Following
We used the below logic when our robot detected an interestion, which is when our robot detects that all line sensors are white. When our robot does not detect an intersection, it line follows as normal.
- If there is no wall to the right, turn right
- If there is a wall to the right, but no wall in front, keep going straight
- If there are walls to the right and in front, but no wall to the left, turn left
- If there are walls on all three sides, turn around
Below is the code used for right hand wall following, using the IR sensor read code from before:
void loop() { readM = analogRead(lineMiddle); readL = analogRead(lineLeft); readR = analogRead(lineRight); float volts1 = analogRead(irM)*0.0048828125; // value from ir sensor int distanceM = 13*pow(volts1, -1); // worked out from datasheet graph float volts2 = analogRead(irR)*0.0048828125; // value from ir sensor int distanceR = 13*pow(volts2, -1); // worked out from datasheet graph float volts3 = analogRead(irL)*0.0048828125; // value from ir sensor int distanceL = 13*pow(volts3, -1); // worked out from datasheet graph // intersection, go right if no wall if (readM < 450 && readR < 450 && readL < 450 && distanceR > wall) { delay(600); turnRight(); } // intersection, wall on the right but no wall straight, go straight else if (readM < 450 && readR < 450 && readL < 450 && distanceM > wall && distanceR <= wall) { straight(); } // intersection, wall in front AND wall to the right, but no wall to the left, turn left else if (readM < 450 && readR < 450 && readL < 450 && distanceM <= wall && distanceR <= wall && distanceL > wall) { delay(600); // remove the delay if sensors are moved near the wheels; otherwise find a good delay time turnLeft(); } // intersection, wall in front AND wall to the right AND to the left, turn around else if (readM < 450 && readR < 450 && readL < 450 && distanceM <= wall && distanceR <= wall && distanceL <= wall) { delay(600); // remove the delay if sensors are moved near the wheels; otherwise find a good delay time turnLeft(); turnLeft(); //turn twice to completely turn around } // intersection, turn right else if (readM < 450 && readR < 450 && readL < 450) { turnRight(); } // not an interection, go straight/line correct else{ lineCorrect(readM, readL, readR); }The robot uses IR sensors in front, on the left, and on the right of the robot to detect walls
We made modifications to the code by playing with the IR sensor range threshold and limiting delays in the code to make the right-hand following more accurate. Below is a video of the robot using our improved algorithm where it successfully traverses the maze:
Robot does right-hand following with improved algorithm