Lab 2 - Bluetooth
Objective
Using the Artemis board and a computer, expand basic application for wireless communication over a Generic Attribute(GATT) framework on top of robust BLE stacks.
Materials/Resources
- 1 x SparkFun RedBoard Artemis Nano
- 1 x USB A-to-C cable
- 1 x Bluetooth 4.0 le
- Distribution code
My edited code
Connect to the Artemis Board
After uploading the provided Arduino sketch (ECE_4960_robot), the serial monitor output the above image. The Artemis board also blinked rapidly after reset as shown in the video.
By running python file main.py, I was able to establish a connection with the robot. At times I had some difficulty connecting the robot, but running main.py several times solved the problem.
Ping your robot
In main.py under myRobotTasks(), I commented out pass and uncommented theRobot.ping(). I ran the main.py to get the output of string ping pong in the serial monitor and the round-trip latency in the command window as seen in the above video.
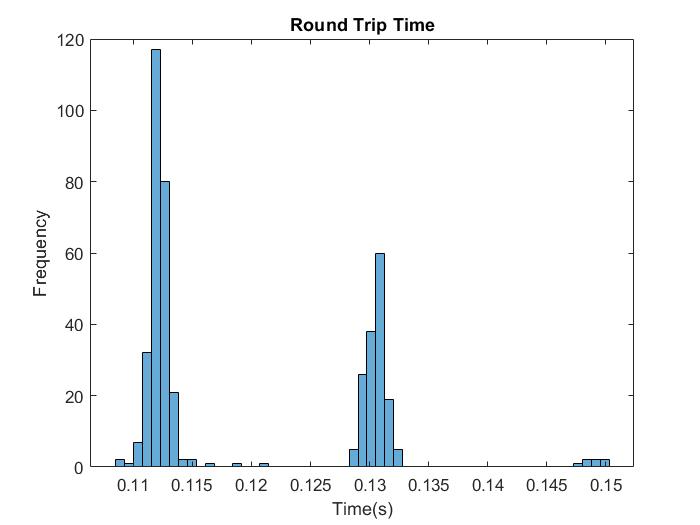
I gathered 427 data points of round-trip times and created a histogram. Interestingly, the data showed bi-modal distribution, indicating that the round-trip time varies mostly between approximately 0.11 and 0.13 seconds. The average of all the data points was 0.1194 seconds. Under command.h, you can see that each ping transfers 99 bytes (one for length, one for command type and 97 for data). Given the bytes and average round-trip latency, I found that the transfer rate is approximately 13,266 bits per second. This is significantly smaller than the serial connection rate of 115,200 bits per second.
Request a float
To request a float, I uncommented theRobot.sendCommand(Commands.REQ_FLOAT) in myRobotTasks() function in main.py and commented out rest of the function definition. In the Arudino sketch, I edited Arduino sketch (ECE_4960_robot) in case REQ_FLOAT to output float value of 5.1. As seen in the image below, the value between input and the output is slightly different. While the value difference may seem negligible, it shows bluetooth connections are not completely accurate when transmitting float values.
Test the Data Rate
To test the data rate of the Artemis board, I first added the code into the Arduino sketch as seen below. I also changed the value bytestream_active to equal 1 in case START_BYTESTREAM_TX. In main.py, I added if (code == Commands.BYTESTREAM_TX.value): print(unpack("<Iqq",data))
and await theRobot.sendCommand(Commands.START_BYTESTREAM_TX)
under myRobotTasks().
if (bytestream_active)
{
res_cmd->command_type = BYTESTREAM_TX;
res_cmd-> length = 70;
uint32_t num1 = 15;
uint64_t num2 = 123456;
uint64_t num3 = 34567;
memcpy(res_cmd->data, &num1, 4);
memcpy(res_cmd->data+4, &num2, 8);
memcpy(res_cmd->data+12, &num3, 8);
amdtpsSendData((uint8_t *)res_cmd, 70);
unsigned long time = micros();
Serial.printf("Count: %d , Time: %d \n", counter, time);
counter ++;
}
When I ran the code for 14 byte and 70 byte packages, I go the following terminal and serial monitor displays:
I collected two different data sets, one sending 14 bytes and another sending 32 bytes. The histograms of the transfer time can be seen below.
For the instance with 14 bytes, average time between packets was 10903 microseconds. Out of 927 packets, 287 were dropped, resulting in a 31% loss.
For the instance with 70 bytes, average time between packets was 10961 microseconds. Out of 700 packets, 343 were dropped, resulting in a 49% loss.
As seen from the data, larger bytes resulted in larger percentage loss of packets, but did not significantly affect the average time between packets.